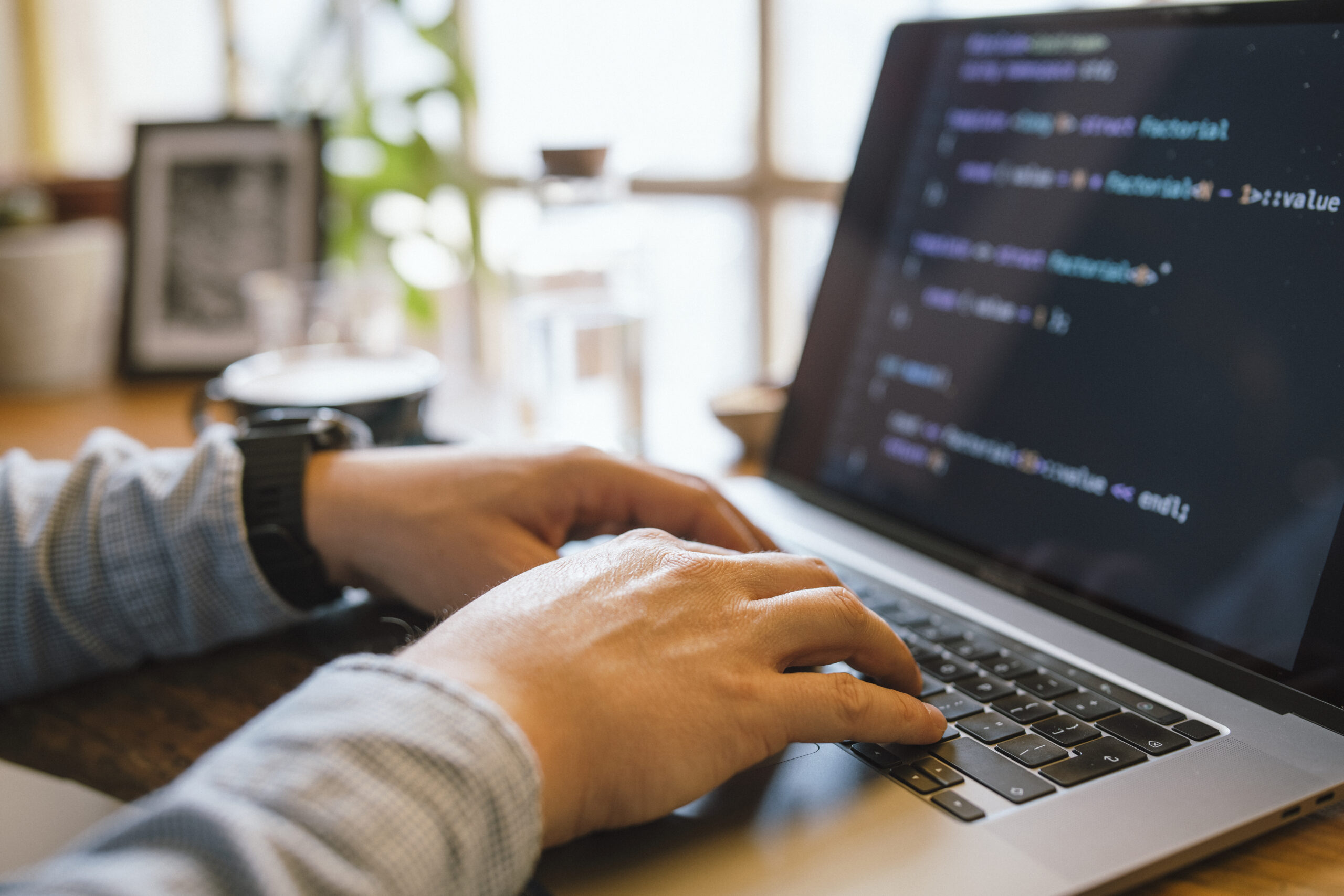
Debugging is Just about the most critical — yet usually neglected — competencies inside of a developer’s toolkit. It's not just about repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to resolve challenges successfully. Irrespective of whether you're a beginner or a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and drastically boost your productivity. Listed here are various approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Tools
One of the fastest approaches developers can elevate their debugging skills is by mastering the applications they use each day. While crafting code is just one Section of advancement, understanding the best way to interact with it proficiently for the duration of execution is equally vital. Modern-day advancement environments appear equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these resources can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code over the fly. When utilised appropriately, they Permit you to observe just how your code behaves in the course of execution, which can be a must have for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclusion developers. They allow you to inspect the DOM, watch network requests, look at real-time functionality metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can convert irritating UI troubles into workable duties.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Handle about running procedures and memory administration. Studying these instruments can have a steeper learning curve but pays off when debugging functionality problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Edition Management devices like Git to understand code historical past, come across the precise moment bugs had been introduced, and isolate problematic alterations.
Ultimately, mastering your resources implies heading over and above default options and shortcuts — it’s about producing an personal knowledge of your improvement surroundings in order that when troubles occur, you’re not dropped in the dead of night. The better you know your tools, the more time you can spend solving the particular issue as opposed to fumbling by means of the method.
Reproduce the situation
The most essential — and sometimes forgotten — techniques in powerful debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, developers have to have to make a regular surroundings or scenario where by the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of prospect, generally resulting in squandered time and fragile code changes.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire questions like: What actions led to The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
As you’ve collected more than enough data, try to recreate the situation in your local natural environment. This might mean inputting precisely the same information, simulating very similar user interactions, or mimicking technique states. If the issue appears intermittently, think about producing automatic exams that replicate the sting cases or condition transitions included. These exams don't just assist expose the situation but also avoid regressions Down the road.
Occasionally, The difficulty may be surroundings-precise — it might take place only on specified functioning methods, browsers, or less than specific configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It needs persistence, observation, and also a methodical solution. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible scenario, You should use your debugging equipment far more proficiently, exam potential fixes safely, and connect more Obviously using your staff or people. It turns an summary grievance into a concrete problem — and that’s the place builders thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when one thing goes Incorrect. Instead of seeing them as frustrating interruptions, builders really should study to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, in which it happened, and occasionally even why it transpired — if you know the way to interpret them.
Commence by studying the information meticulously As well as in total. Many builders, especially when under time force, glance at the main line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the mistake down into areas. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Mastering to recognize these can dramatically hasten your debugging procedure.
Some faults are vague or generic, and in All those cases, it’s critical to look at the context in which the error transpired. Check relevant log entries, enter values, and recent alterations in the codebase.
Don’t neglect compiler or linter warnings both. These frequently precede larger sized concerns and provide hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint troubles a lot quicker, reduce debugging time, and become a much more effective and assured developer.
Use Logging Correctly
Logging is Among the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, serving to you fully grasp what’s happening under the hood without having to pause execution or move from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through improvement, INFO for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t split the applying, ERROR for actual complications, and Deadly once the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant facts. An excessive amount logging can obscure critical messages and slow down your procedure. Target important events, condition modifications, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments exactly where stepping by code isn’t feasible.
Additionally, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a well-imagined-out logging tactic, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your code.
Feel Just like a Detective
Debugging is not merely a technological job—it's a sort of investigation. To correctly determine and resolve bugs, developers should strategy the method similar to a detective resolving a secret. This mindset assists break down complex problems into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering proof. Look at the symptoms of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, accumulate just as much suitable facts as you may without jumping to conclusions. Use logs, test cases, and person experiences to piece jointly a transparent photo of what’s taking place.
Up coming, sort hypotheses. Question by yourself: What may be leading to this conduct? Have any modifications recently been built to your codebase? Has this challenge happened in advance of beneath equivalent situations? The goal should be to slim down prospects and determine opportunity culprits.
Then, take a look at your theories systematically. Try to recreate the situation in the controlled ecosystem. When you suspect a particular function or ingredient, isolate it and confirm if The problem persists. Like a detective conducting interviews, request your code concerns and Enable the outcome lead you nearer to the truth.
Fork out near attention to smaller facts. Bugs usually disguise from the least envisioned areas—similar to a missing semicolon, an off-by-one error, or a race condition. Be complete and individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short term fixes may perhaps conceal the actual issue, just for it to resurface later.
And lastly, maintain notes on That which you attempted and figured out. Just as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and help Other folks comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in advanced more info systems.
Create Assessments
Producing checks is among the most effective approaches to transform your debugging competencies and All round development efficiency. Tests not just support capture bugs early but will also function a security Web that offers you confidence when creating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Start with unit exams, which concentrate on personal functions or modules. These tiny, isolated exams can rapidly reveal whether or not a specific bit of logic is Doing the job as expected. When a exam fails, you straight away know where by to glimpse, noticeably cutting down enough time put in debugging. Unit tests are Particularly beneficial for catching regression bugs—troubles that reappear soon after Formerly getting set.
Subsequent, combine integration assessments and conclude-to-finish tests into your workflow. These assistance be sure that various aspects of your application perform together efficiently. They’re specifically helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If some thing breaks, your checks can let you know which part of the pipeline failed and under what problems.
Creating checks also forces you to Imagine critically about your code. To check a attribute effectively, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a difficulty, creating a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and enjoy your test move when The difficulty is fixed. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch much more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, striving Option just after Answer. But Among the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your thoughts, minimize disappointment, and sometimes see The problem from a new point of view.
When you are also near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code you wrote just hrs previously. On this state, your brain becomes fewer effective at problem-resolving. A brief stroll, a coffee crack, or simply switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of an issue when they've taken time and energy to disconnect, allowing their subconscious perform within the history.
Breaks also enable avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You would possibly abruptly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you right before.
In case you’re stuck, a superb guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially less than tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart method. It presents your brain Room to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you encounter is much more than just a temporary setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you one thing precious for those who make an effort to mirror and assess what went Completely wrong.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots as part of your workflow or knowledge and assist you Establish much better coding behaviors transferring forward.
Documenting bugs can be a superb behavior. Maintain a developer journal or preserve a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see patterns—recurring problems or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts staff effectiveness and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from stress to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your progress journey. In any case, a number of the most effective developers are usually not the ones who produce ideal code, but people that constantly study from their mistakes.
In the long run, each bug you correct provides a brand new layer on your talent set. So following time you squash a bug, have a moment to mirror—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become improved at what you do.