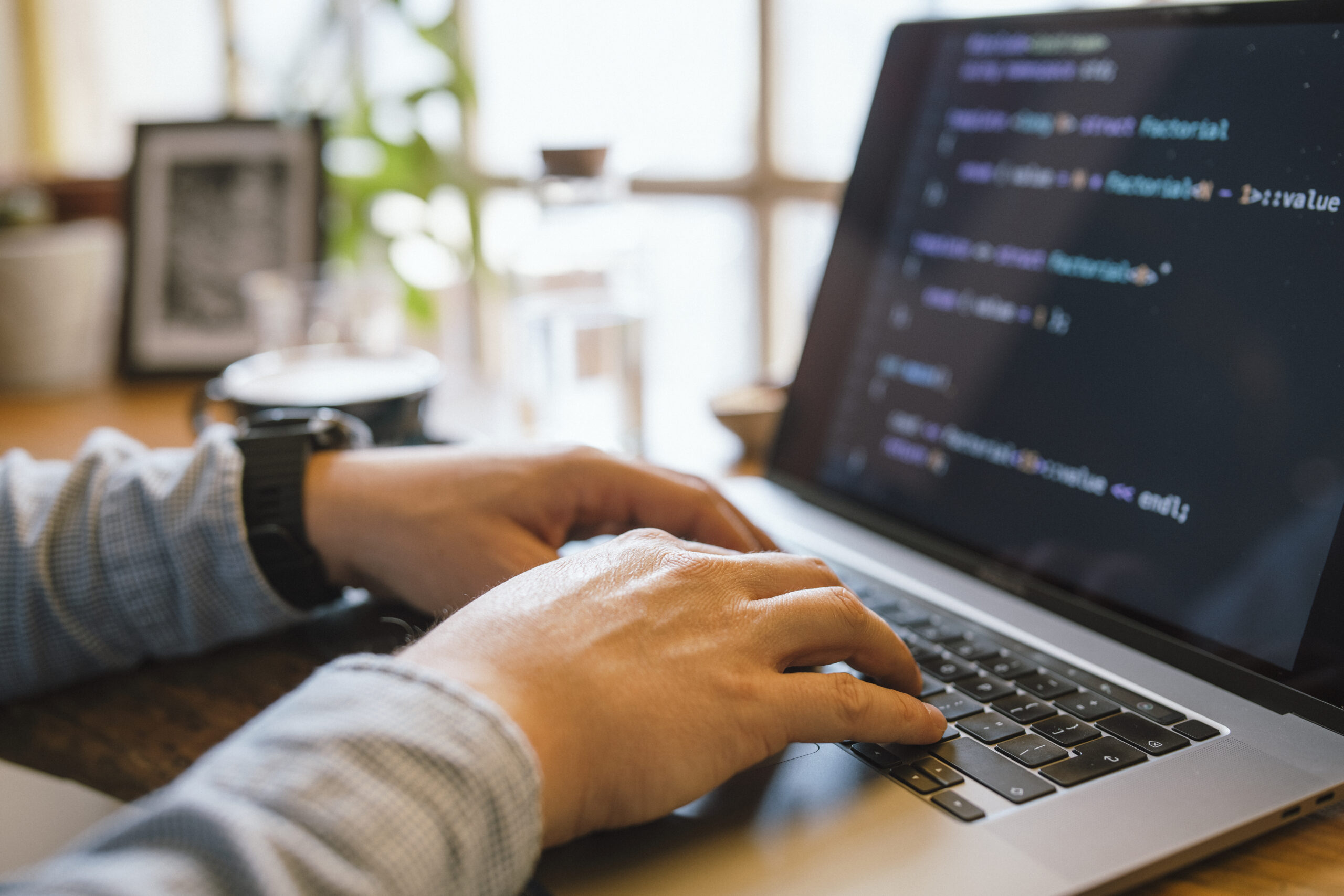
Debugging is One of the more crucial — still normally overlooked — abilities within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Feel methodically to solve difficulties proficiently. No matter whether you're a novice or even a seasoned developer, sharpening your debugging expertise can preserve hrs of disappointment and drastically boost your productivity. Listed here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is a single A part of development, recognizing tips on how to communicate with it effectively all through execution is Similarly essential. Modern progress environments arrive Outfitted with impressive debugging abilities — but a lot of developers only scratch the area of what these instruments can perform.
Acquire, by way of example, an Integrated Enhancement Natural environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, action by way of code line by line, as well as modify code over the fly. When utilised correctly, they Enable you to observe accurately how your code behaves for the duration of execution, which can be a must have for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclude developers. They enable you to inspect the DOM, monitor network requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can change discouraging UI problems into manageable responsibilities.
For backend or method-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper Studying curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, grow to be relaxed with Variation control methods like Git to grasp code heritage, obtain the precise moment bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when problems arise, you’re not misplaced at midnight. The better you realize your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
One of the more important — and sometimes neglected — measures in efficient debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
As you’ve collected sufficient information and facts, make an effort to recreate the problem in your local environment. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, take a look at probable fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance right into a concrete problem — and that’s exactly where developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as aggravating interruptions, developers should master to deal with error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in full. Quite a few developers, especially when underneath time stress, look at the 1st line and right away start building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally stick to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in those circumstances, it’s important to look at the context by which the error happened. Check associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it provides true-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method starts with understanding what to log and at what level. Common logging levels include DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic information during enhancement, Facts for standard functions (like productive begin-ups), WARN for opportunity difficulties that don’t split the application, Mistake for true difficulties, and FATAL in the event the technique can’t proceed.
Steer clear of flooding your logs with too much or irrelevant facts. A lot of logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, state changes, enter/output values, and critical conclusion details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a properly-assumed-out logging strategy, you may reduce the time it will take to identify challenges, acquire deeper visibility into your purposes, and improve the All round maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological task—it's a type of investigation. To proficiently detect and repair bugs, developers have to tactic the procedure similar to a detective solving a mystery. This attitude will help stop working advanced challenges into workable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much relevant info as you are able to with out jumping to conclusions. Use logs, test cases, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Up coming, type hypotheses. Request your self: What might be leading to this conduct? Have any modifications recently been made into the codebase? Has this challenge transpired just before below similar instances? The target is usually to narrow down possibilities and detect probable culprits.
Then, take a look at your theories systematically. Try and recreate the issue in a managed surroundings. In the event you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs frequently cover inside the the very least anticipated sites—just like a lacking semicolon, an off-by-a single mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes may conceal the actual issue, just for it to resurface afterwards.
Finally, retain notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for potential challenges and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in complex methods.
Publish Assessments
Crafting tests is one of the most effective strategies to transform your debugging competencies and overall advancement effectiveness. Assessments not simply enable capture bugs early but will also function a security Web that offers you confidence when creating modifications in your codebase. A effectively-examined application is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Get started with device checks, which center on unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a certain piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Unit checks are Specially valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These enable be certain that numerous parts of your software perform together efficiently. They’re specifically useful for catching bugs that come about in intricate systems with various elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and beneath what circumstances.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—staring at your display for hours, striving Resolution immediately after Alternative. But Probably the most underrated debugging resources is just stepping away. Taking breaks aids you reset your head, cut down irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this state, your brain results in being fewer economical at challenge-fixing. A short walk, a espresso split, and even switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a difficulty once they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something valuable should you make time to mirror and review what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught before with better practices like unit tests, code reviews, or logging? The responses often expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding others steer clear of the identical issue boosts staff effectiveness and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from stress to curiosity. Rather than dreading Gustavo Woltmann coding bugs, you’ll get started appreciating them as vital parts of your progress journey. In the end, a lot of the greatest builders usually are not those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer in your talent established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Bettering your debugging competencies requires time, follow, and tolerance — but the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.